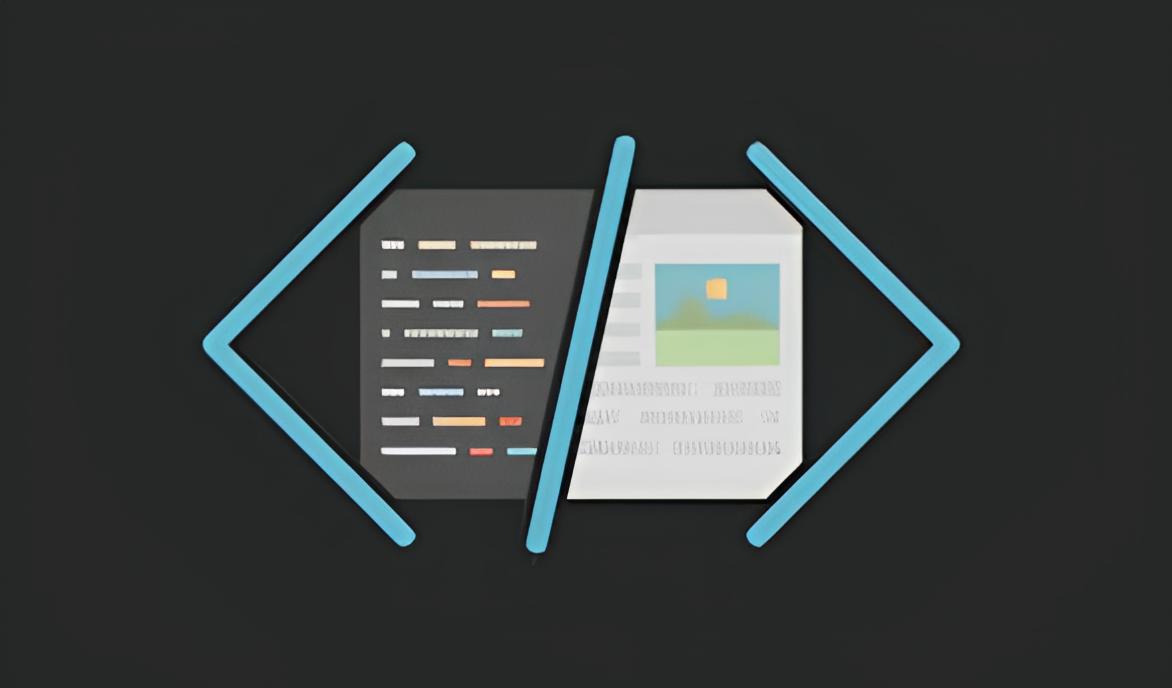
基于 React 实现将HTML页面内容转化成图片,并支持下载
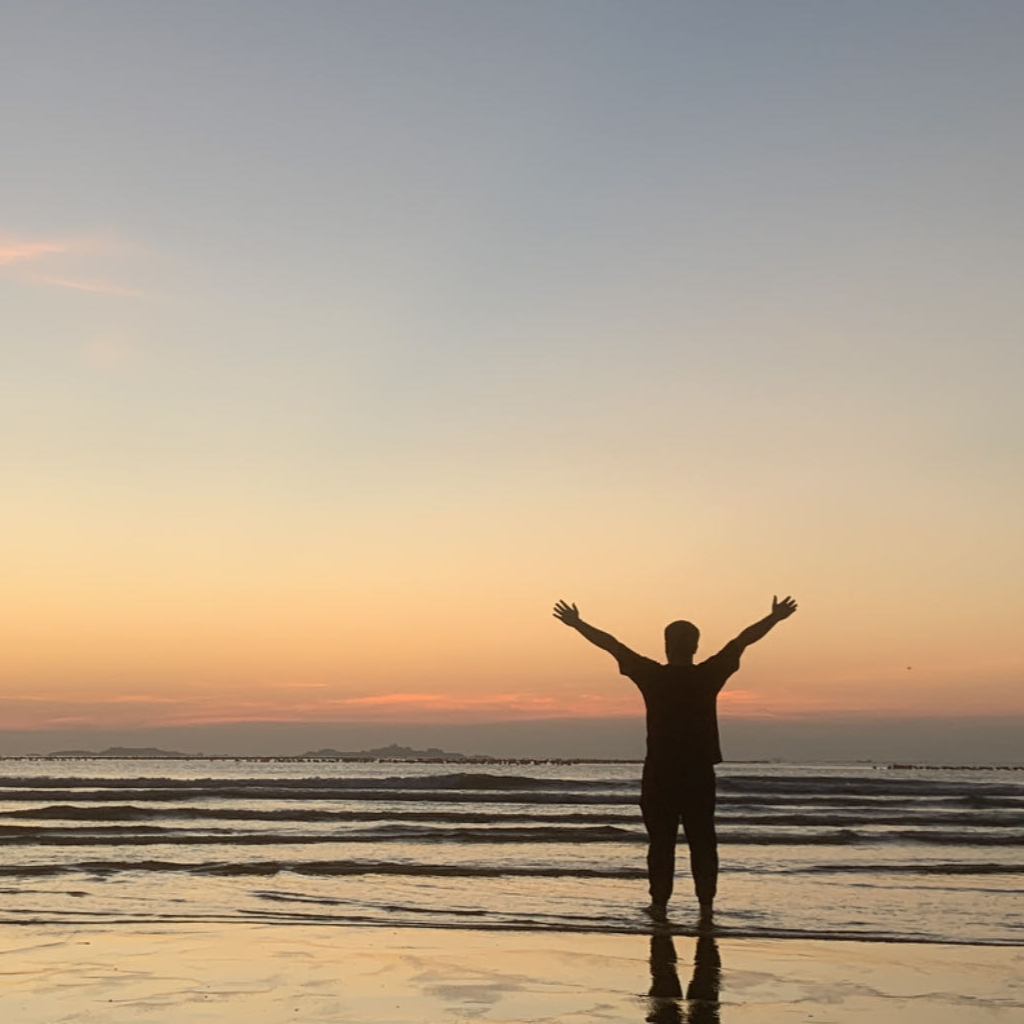
前言
在Web开发中,有时我们希望将网页内容或某个特定的区域转换成图片格式,以便用户可以下载。比如,这在生成报表截图、分享社交媒体帖子等场景下非常有用。
在本教程中,将引导您如何使用这两个库来捕获网页内容,并让用户可以将其作为图片下载。
使用html2canvas
一、安装依赖
通过npm或yarn安装 html2canvas
:
bashnpm install html2canvas # 或者 yarn add html2canvas
二、实现组件
jsximport React from 'react'; import html2canvas from 'html2canvas'; const DownloadWithHtml2Canvas = () => { const downloadAsImage = async () => { const element = document.getElementById('capture-area'); const canvas = await html2canvas(element); const image = canvas.toDataURL("image/png", 1.0); const link = document.createElement('a'); link.href = image; link.download = 'download.png'; document.body.appendChild(link); link.click(); document.body.removeChild(link); }; return ( <> <div id="capture-area" style={{ padding: '20px', backgroundColor: 'white' }}> <h1>Hello, World!</h1> <p>Here is some content we want to capture as an image.</p> </div> <button onClick={downloadAsImage}>Download as Image</button> </> ); }; export default DownloadWithHtml2Canvas;
在此组件中,我们使用html2canvas将指定的DOM元素(#capture-area
)转换为一个Canvas元素,然后将Canvas转换为数据URL并触发下载。
使用html-to-image
一、安装依赖
通过npm或yarn安装 html-to-image
:
bashnpm install html-to-image # 或者 yarn add html-to-image
同时,我们将使用 file-saver
来协助下载图片:
bashnpm install file-saver # 或者 yarn add file-saver
二、实现组件
jsximport React from 'react'; import * as htmlToImage from 'html-to-image'; import { saveAs } from 'file-saver'; const DownloadWithHtmlToImage = () => { const downloadAsImage = async () => { try { const dataUrl = await htmlToImage.toPng(document.getElementById('capture-area')); saveAs(dataUrl, 'download.png'); } catch (error) { console.error('Oops, something went wrong!', error); } }; return ( <> <div id="capture-area" style={{ padding: '20px', backgroundColor: 'white' }}> {/* Your content goes here */} <h1>Hello, World!</h1> <p>Here is some content we want to capture as an image.</p> </div> <button onClick={downloadAsImage}>Download as Image</button> </> ); }; export default DownloadWithHtmlToImage;
在这个组件中,html-to-image
的 toPng
方法被用来将DOM元素转化为PNG图片。一旦转换成功,我们利用 file-saver
的 saveAs
方法来保存图片。
两种方法的对比
html2canvas
是一个更加成熟的库,它提供了广泛的社区支持,但可能在渲染一些复杂CSS样式时遇到困难。html-to-image
是一个较新但轻量级的库,它提供了额外的图片格式(如SVG)支持,并可能在处理特定元素(如动态Canvas)时表现更好。
总结
现在您已经知道如何在React应用中将HTML内容转换成图片,并实现了一个下载功能。这样,用户就可以方便地将网页的某,部分内容保存为图片,从而用于报告、展示或分享。
请记住,无论您选择哪种库,都可能存在兼容性和性能的考量。特别是在大型DOM结构或复杂CSS样式的情况下。在将功能投入生产环境之前,确保进行充分的测试,以得到最佳的用户体验。